|
1 | 1 | [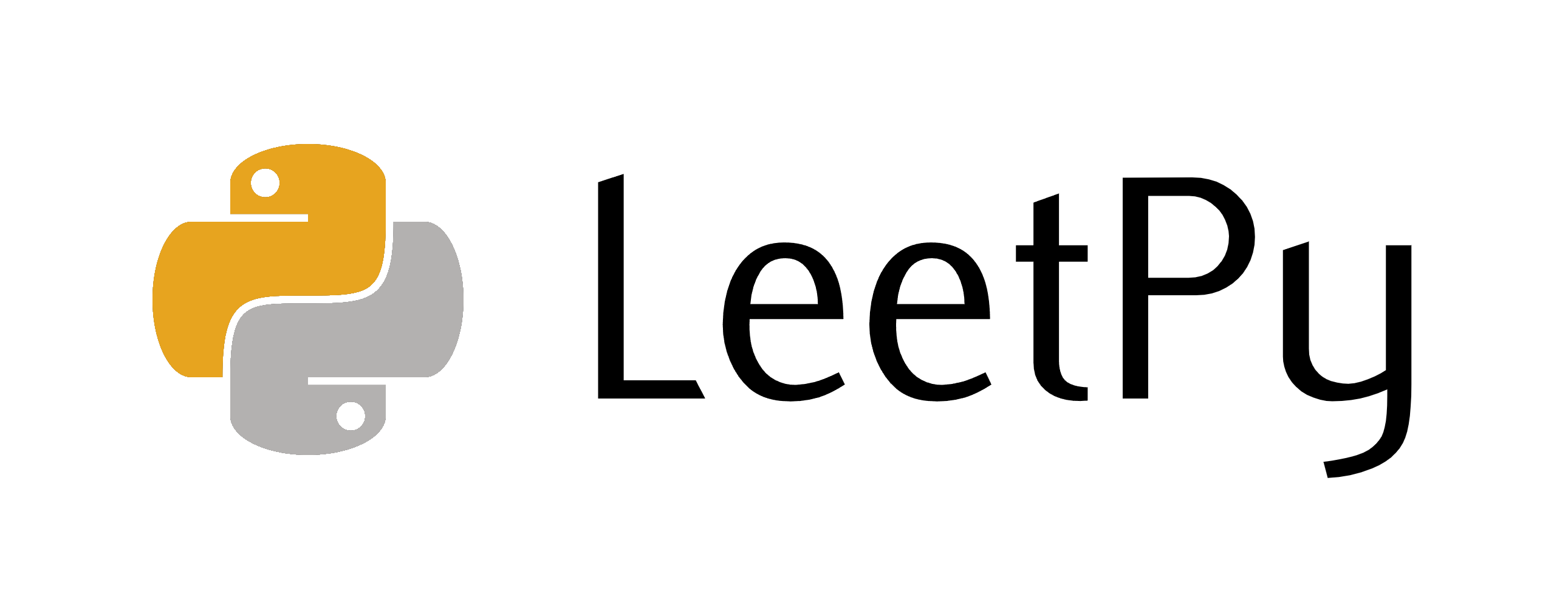](https://github.com/aryanpingle/LeetPy)
|
2 | 2 |
|
3 |
| -The ultimate tool to have while solving Leetcode questions. |
| 3 | +> Debugging is twice as hard as writing the code in the first place. |
| 4 | +
|
| 5 | +If you solve problems related to Data Structures & Algorithms (DSA), you know how frustrating it is to debug complex data structures like Binary Trees and Directed Graphs. |
| 6 | + |
| 7 | +**`LeetPy`** is a lightweight Python package that makes you more efficient when you solve DSA problems. It contains utility functions and algorithms that make debugging and testing *SO MUCH* easier. Here are some features: |
| 8 | + |
| 9 | +- **Several Data Structures**: Binary Trees, Linked Lists, 2-D Arrays, etc. |
| 10 | +- **Visualize Your Objects**: **`LeetPy`** provides convenient `print()` functions that show you what your structure looks like (all inside your terminal!). |
| 11 | +- **Save & Export**: Serialization and export functions help you save an exact copy of your structure, and give you the code to generate them from scratch. |
| 12 | +- **Flexibility**: Create your data structure *however* you want; **`LeetPy`**'s algorithms will always work correctly. |
| 13 | + |
| 14 | +## Usage & Examples |
| 15 | + |
| 16 | +Consider debugging the Binary Tree data structure: |
| 17 | + |
| 18 | +```python |
| 19 | +from leetpy import BinaryTree |
| 20 | + |
| 21 | +# from leetpy import TreeNode |
| 22 | +class TreeNode: |
| 23 | + """A basic definition of a binary tree's node.""" |
| 24 | + |
| 25 | + def __init__( |
| 26 | + self, |
| 27 | + val: any = 0, |
| 28 | + left: Optional["TreeNode"] = None, |
| 29 | + right: Optional["TreeNode"] = None, |
| 30 | + ): |
| 31 | + self.val = val |
| 32 | + self.left = left |
| 33 | + self.right = right |
| 34 | + |
| 35 | +# Randomly generate the root of a binary tree |
| 36 | +root: TreeNode = BinaryTree.create(n=20, min_val=1, max_val=10) |
| 37 | +# Or, import it from a serialized array (like on Leetcode) |
| 38 | +root: TreeNode = BinaryTree.create_from_leetcode_array("[1,2,3,4,null,null,5]") |
| 39 | + |
| 40 | +# Count the number of nodes in the binary tree |
| 41 | +print(BinaryTree.count_nodes(root)) |
| 42 | +# Print all the nodes of the binary tree |
| 43 | +for node in BinaryTree.travel_inorder(root): |
| 44 | + print(node) |
| 45 | +# Visualize the structure of the binary tree |
| 46 | +BinaryTree.print_structure(root) |
| 47 | +``` |
| 48 | + |
| 49 | +**`LeetPy`** offers a wide range of utility functions - for a wide range of data structures. |
| 50 | + |
| 51 | +## Installation |
| 52 | + |
| 53 | +To install the latest stable release, run: |
| 54 | + |
| 55 | +```bash |
| 56 | +$ pip install leetpy |
| 57 | +``` |
| 58 | + |
| 59 | +To install from the latest GitHub commit: |
| 60 | + |
| 61 | +```bash |
| 62 | +pip install git+https://github.com/aryanpingle/leetpy |
| 63 | +``` |
0 commit comments